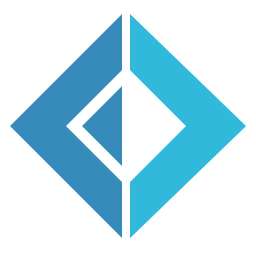
In our F# code, we have a lot of asynchronicity: query the DB, call external services, write messages to the service bus, etc. Inside of our happy F# bubble we use async workflows, either by using Async or by using the computation expression async { ... }. But we are surrounded by a world of Tasks. So we often need to call functions or methods that return a Task. Of course, that is no big problem, we can just add |> Async.AwaitTask, but that leads to a lot of visual clutter in our precious...